The film critic Roger Ebert states they are not, and can never be. Ignoring the fact that he doesn’t play games himself, so couldn’t possibly experience any engagement that might indicate whether he’s right or not, one of his main points is that their interactive nature precludes videogames from ever being art. Could a game of Chess be considered art, he asks.
I think by making this assertion he’s actually missed something quite important, and to illustrate this, I have an example.
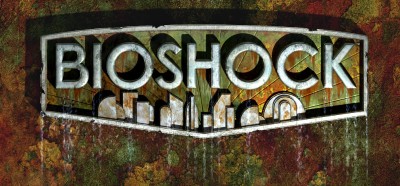
In the game Bioshock, your character is exploring a beautiful art-deco underwater city, having pitched up there after a plane crash. Note: This will contain major spoilers for the game. Please don’t continue if you haven’t played it and intend to at some time – the revelations are worth discovering on your own.
As you explore you (and the character you’re controlling) learn more about the city’s backstory, about the mysterious Andrew Ryan, who created the city, and about his social and biochemical attempts to create a perfect city.
The visual look of the game is fantastic, and wouldn’t disgrace any hollywood movie, but that’s not the reason I think it qualifies as art. Art isn’t about the quality of the rendering, or many modern artists wouldn’t qualify.
The true art in this game comes from its effect on you, the player (the audience, if you like) but I would argue that the effect it has on you is enhanced, rather than diminished, by the fact it is interactive. Here’s why.
Early on in the game, you meet a creepy little girl, known as a ‘Little Sister’. You learn that she’s been genetically engineered, and she stalks the city looking for dead people from whom she can extract a vital drug. The first time you meet one of these girls you’re told several things by the character who’s guiding your progress through the game:
- They aren’t really little girls, because they’ve been enetically engineered
- The drug they extract is something you need to improve your abilities within the game
- To get hold of that drug you have to kill the little girl
At the same time, another character who you’ve never met before implores you not to harm the girl. She tells you:
- You don’t have to kill her – you can ‘rescue’ her which frees her from the need to extract the drug
- Rescuing her will still give you some of the drug she’s carrying, but not as much
- If you rescue them, she will make sure you’re rewarded later (although she doesn’t specify how)
So as a player you have a clear choice: Kill what looks like a little girl for an instant reward, or rescue her, and hope that your future reward is worth it. Your ability to progress in the game wight well be affected by your decision – the game involves various weapons and abilities, some of which can become more powerful the more of the drug you get.
Now obviously, this is a game, a fairly violent one where you’ve already been killing plenty of other characters (think of ‘fast zombies’ from 28 days later for the kinds of enemies you have to kill) but this choice your given is very different. The game designers have very deliberately chosen to make these characters little girls, then offered you this choice of ways to play the game. (I don’t think you can also choose to do neither – to move past this point in the game, I think you have to choose, but later in the game you could choose to ignore the girls. But you have to make the choice at least once).
So as you play the rest of the game, each time you encounter a little sister, you make the same choice. When I played it, I always chose to rescue them.
The important thing here is that your choice has a profound effect on the ending of the game, which I’ll come to in a moment.
Much later in the game, there is a significant twist. You confront Andrew Ryan, and he reveals that the character you are playing as was actually born in the city, and was psychologically engineered to be controlled by a key phrase, and has been controlled by the character in the game who has, up to this point, been guiding you around the city, telling you where to go and what to do.
Then Ryan orders you to kill himself. With a golf club.
Which you do.
This is a fairly shocking moment, but interestingly, one over which you have no choice. The game is in a ‘cut scene’ at this point, and you have no control over what happens. Andrew Ryan is killed, by you, and you can’t stop it happening.
The first time I played this I thought I’d acidentally hit the ‘Fire’ button, and was horrified. I reloaded the game from an earlier point to check, but it all still happened the same way.
This is the first point in the game where I think the fact it’s a game makes it art. We’re used to killing or destroying things and people in games. But at this point, the designers chose not to give you a choice. The narrative happens regardless, and yet you feel shocked and culpable. This is a reaction which is amplified by it being a game. If you were seeing the same story play out in a linear form, it would still be a shocking moment, but your emotional reaction to it would be less. Or at least, different.
This is an example of being art because it’s a game, not despite.
And the second example, from the same game, is the ending.
Having killed Ryan, your game character is eventually freed from the psychological influence of Ryan’s nemesis, Frank Fontaine, and the last section requires you to fight him and kill him. Frankly, this is a typical ‘End of game Boss Level’ – you confront someone stronger than anyone you’ve previously encountered, and have to defeat them to complete the game. This is a convention of video games, almost like the ride into the sunset in cowboy movies, and is nothing remarkable until you finally overpower him. You then get the endgame.
And it’s different, depending on that choice you made early in the game. If you chose to rescue the little sisters, the game shows you one ending. After they’ve helped you kill Fontaine, one of the sisters approaches you, handing you something, and the following narration is spoken by the female doctor who looked after the sisters:
They offered you their city.
And you refused it.
And what did you do instead?
What I have come to expect of you.
You saved them.
You gave them the one thing that was stolen from them: A chance.
A chance to learn. To find love. To live.
And in the end what was your reward?
You never said, but I think I know.
A family.
It’s quite beautiful. I’m not ashamed to say, I cried at that ending. Someone once said that games could not be classed as art until you truly care about the characters, in which case, job done.
But I think this offers something slightly deeper. Because this ending is a direct response to your behaviour during the game. You only get this ending if you rescued the little sisters. You get a different ending (see previous link) if you harvested them, a far more prosaic ending (in my view).
But the truly interesting thing is that that happy ending is your ‘reward’. You’ve earned it. The game gives you this emotional response because of the way you’ve played it. No linear art form could do this – it’s only possible because a game is a thing to be played.
And that is why games can truly be art. Because they have a wholly unique way to affect you.